Tackling the Tricky Bits in Coding: What’s the Hardest Part?
Posted on Feb 20, 2025 by Elara Greenfield
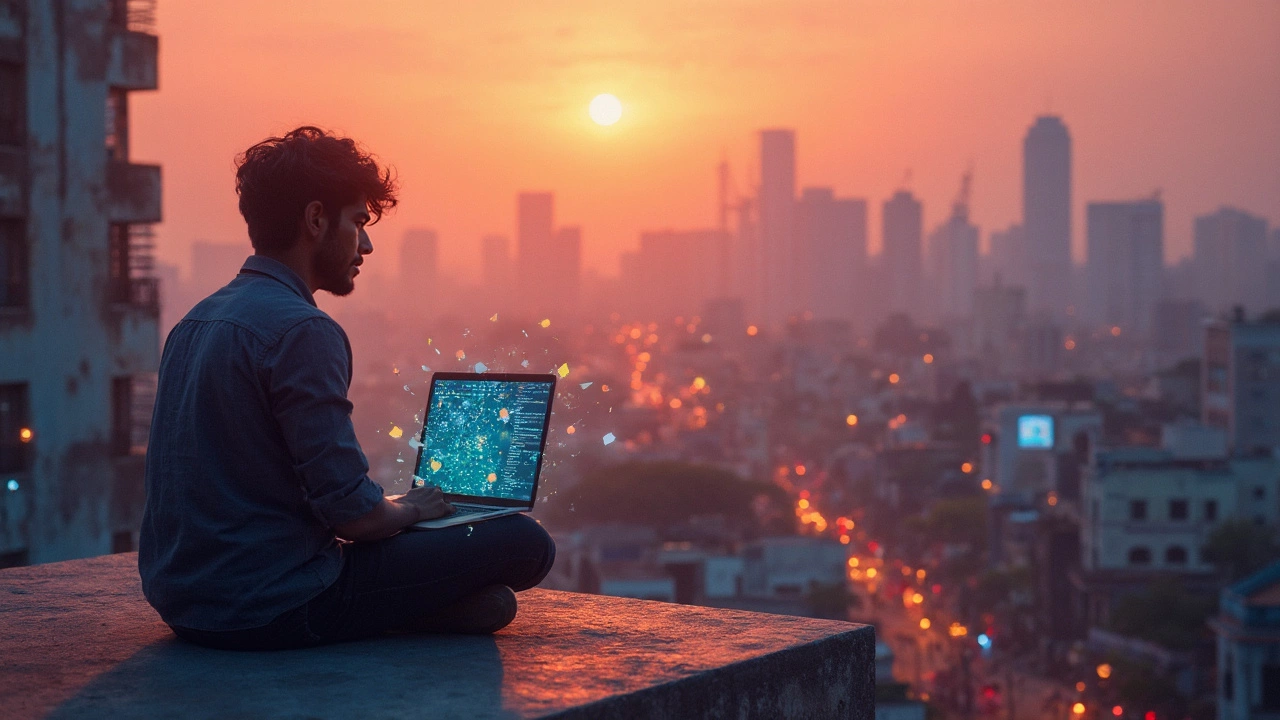
If you're just starting out with coding, welcome to the wild ride of learning a new language—not the kind you're used to, but one filled with symbols, loops, and logic. The whole thing can feel like trying to learn to read music when you've never even hummed a tune. One of the hardest parts? Debugging. It’s the coding equivalent of playing detective, hunting down those pesky errors that stop your program in its tracks. It might surprise you, but even seasoned developers get sweats when they're deep in a debugging session.
Another brain-buster is algorithms. They're the backbone of coding, those step-by-step procedures that solve specific problems. They can seem like a foreign concept at first—an abstract puzzle that needs visualization before you can even start thinking about solutions. For beginners, imagining how an algorithm flows can be downright baffling. But hang in there, because breaking them down into smaller parts is key, transforming confusion into clarity.
- The Frustration of Debugging
- Understanding Algorithms
- Conceptualizing Data Structures
- Mastering Syntax and Semantics
- Overcoming Imposter Syndrome
The Frustration of Debugging
Ah, debugging—a coder’s nemesis. It’s like finding a needle in a haystack, except the haystack is on fire and someone’s removed all the needles. Debugging can make even the most seasoned developers scratch their heads in confusion. But fear not, it’s all part of the coding journey. The key is understanding what debugging really involves and how you can get better at it.
Identifying Bugs
The first step in debugging is spotting the problem. This sounds simple, but in reality, it can be the toughest part. Sometimes, an issue doesn't break the code immediately; it just makes things act… weird. For example, your program might run, but produce incorrect results. You need to figure out where it’s going wrong, which means paying close attention to the code’s behavior and output.
Using tools like breakpoints and console logs are essential for spotting where things go off the rails. I mean, who doesn't love a good coding challenge? Visual debuggers let you run your code step-by-step, showing exactly what’s happening at each stage. It’s like having a magic magnifying glass to see what’s really going on.
Common Debugging Strategies
Coding issues can often be divided into three buckets: syntax errors, runtime errors, and logical errors. Here’s a quick rundown of how to tackle each:
- Syntax errors: These are usually the easiest to find because the compiler or interpreter will point them out. Look for any typos or missing symbols in your code.
- Runtime errors: These occur when your program is running. Keeping an eye on your stack trace can give you a clue where the error happened.
- Logical errors: These involve the logic you’ve written being incorrect. These are sneaky because the program runs but doesn’t do what you expect. That’s why testing with lots of different cases is key to catching these.
The Importance of Testing
Testing is your best friend when it comes to debugging. If you test as you go, you’re more likely to catch errors before they spiral out of control. Unit tests check if small parts of your program work individually. It’s like testing each Lego piece before building the whole castle.
Moreover, using version control systems like Git can save you a lot of headaches. You can always go back to a previous working version of your code if things go really south.
Type of Error | Description |
---|---|
Syntax Errors | Typos or missing symbols in code |
Runtime Errors | Occurs during program execution |
Logical Errors | Program runs but with unexpected results |
Remember, debugging is a learning process. Each bug you fix is a lesson learned, making you a stronger programmer. So next time you’re knee-deep in error messages, take a deep breath and remember: you’re not alone, and you’re definitely getting better with each fix.
Understanding Algorithms
Navigating the world of algorithms can feel like a deep dive into uncharted waters, but grasping them is crucial for mastering coding. Algorithms aren't just about solving problems; they're about solving them efficiently. Let's break it down in clearer terms. Imagine you're sorting a stack of shuffled cards. How you approach the sorting is an algorithm—there's more than one way to do it, but some ways are faster than others.
Why Are Algorithms Important?
Algorithms are the framework of coding logic. They tell the computer what to do and in what order. For example, the search algorithm used by Google is what makes its results lightning-fast and relevant. Without a clear understanding of algorithms, writing efficient and effective code becomes a guessing game.
Common Types of Algorithms
Most aspiring coders encounter a few key types early on:
- Sorting Algorithms: These arrange data in a specific order; think Bubble Sort, Quick Sort, and Merge Sort.
- Search Algorithms: Finding elements in a data set is guided by these; Linear Search and Binary Search are staples.
- Recursive Algorithms: They call themselves repeatedly to solve a problem; common in mathematical computations like calculating Fibonacci numbers.
Understanding these helps you choose the right tool for the job, which is crucial because the right algorithm can save significant time and resources.
Steps to Master Algorithms
- Understand the Problem: Before diving into coding, ensure you fully grasp what needs to be solved.
- Start Simple: Begin with basic problems and simple algorithms; build your confidence before tackling complex ones.
- Break It Down: Fragment the algorithm into smaller, digestible parts.
- Practice: Consistent practice is key. Use platforms like LeetCode or HackerRank to apply your knowledge.
Algorithm knowledge is a big milestone in learning to code. It transforms confusion into clarity—making the seemingly impossible, possible.
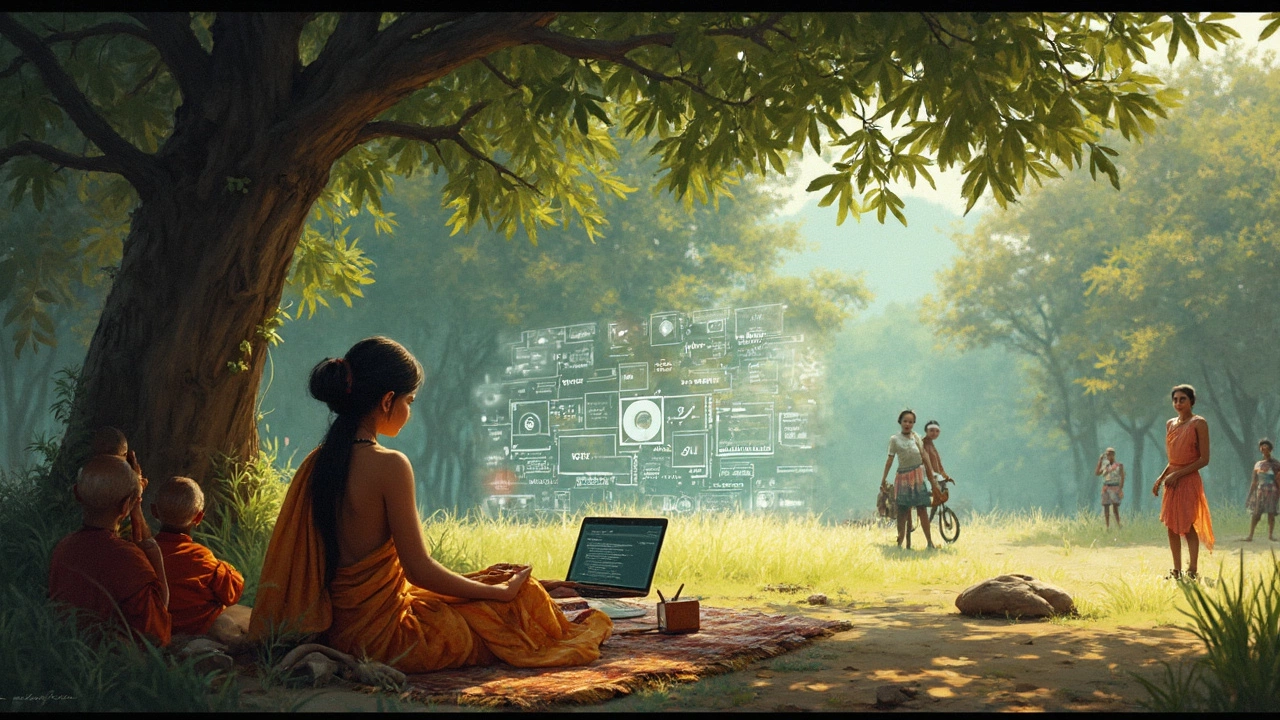
Conceptualizing Data Structures
Ah, data structures—those mysterious containers of code that store and organize data. When you're knee-deep in learning to code, getting your head around data structures can feel like trying to organize a huge Lego set with no instruction guide. But don't worry, it's all about understanding the basic shapes and how they fit together in the world of coding.
In the simplest terms, data structures are like the filing cabinets of programming. They help store your data efficiently so you can retrieve and manipulate it without breaking a sweat. Common examples include arrays, lists, stacks, and queues, each with its own set of rules and use-cases, but they all aim for the same goal: keeping your data tidy.
Why It’s Hard
So, why are data structures one of the biggest coding challenges? Well, initially, you need to choose the right one for your problem. It’s like picking the right tool from a toolbox—kind of hard if you don’t know what each tool does. Beginners often struggle with this selection process because they haven’t yet seen how each data structure operates in different scenarios.
Breaking It Down
- Arrays: Think of these as a chain of boxes, all in a row. Perfect for when you know exactly how much stuff you're storing.
- Linked Lists: A bit like a treasure hunt, where each box has a clue (or pointer) to the next one. Great when your data could change in size.
- Stacks: Imagine a stack of plates. Last one in is the first one out—also known as LIFO (Last In, First Out) operation.
- Queues: Like waiting for rides at an amusement park. First in, first out—just like a fair queue.
Here's a quirky stat: a study showed that computer science students take, on average, three times longer to master data structures compared to syntax basics. That’s because data structures demand you to think logically about organization rather than just syntax—it's about how well you can strategize.
In the end, mastering data structures is a real game-changer in learning to code. Visual aids can be super helpful here. Drawing diagrams of how each structure works or tracing code execution on paper can often lead to those satisfying "ah-ha!" moments. Remember, it's less about memorizing and more about understanding.
Mastering Syntax and Semantics
Diving into coding can feel like entering a whole new world where just a misplaced semicolon can be your worst enemy. Learning the syntax of a programming language is crucial because even the slightest typo can prevent your code from running. Think of syntax as the grammar of coding, and just like learning any new language, it takes practice and patience.
Let’s talk specifics. Every language—be it Python, Java, or JavaScript—has its own unique set of rules. Python, for example, is known for its readability and uses indentation for blocks, whereas Java uses curly braces. Mastering these differences is like knowing when to use different punctuation marks in writing.
Understanding Semantics
Semantics, on the other hand, is about the meaning behind the code. It’s not enough to just write syntactically correct code; your code needs to actually do what you intend. Imagine writing an essay with perfect grammar but no coherent message. That’s what code with the right syntax but poor semantics is like.
Here's a quick tip: commenting your code as you write it can reinforce your understanding of both syntax and semantics. By explaining each step in plain English, you bridge the gap between knowing and doing.
Common Pitfalls and How to Avoid Them
- Confusing data types: Know your data structures. Understand when to use an array versus a list, especially in languages like Python where these differences matter.
- Naming conventions: Stick to meaningful names for your variables and functions. It might seem trivial, but clear naming can make a huge difference in maintaining and understanding your code later.
- Off-by-one errors: This common mistake happens during looping. Understanding zero-based indexing will help you keep these errors in check.
Lastly, remember that learning to code is an iterative process. You’ll make mistakes, but with each error comes a new lesson. The more you code, the more natural both syntax and semantics will become. So, keep coding and don’t be afraid of making errors—each one brings you closer to mastering the coding challenges.
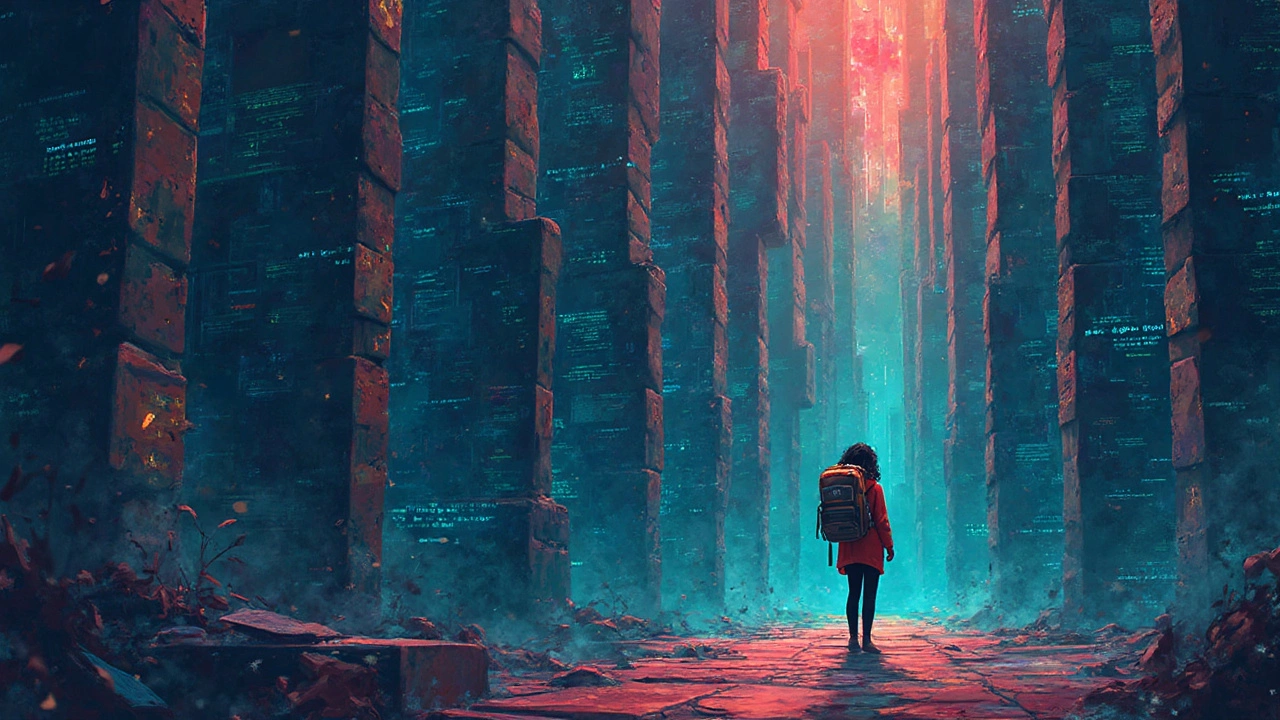
Overcoming Imposter Syndrome
Ever had that nagging feeling like you're faking it and everyone will find out? Welcome to imposter syndrome—a frequent, unwelcome visitor in the coding world. It doesn't matter if you're a newbie in a coding class or a seasoned pro; self-doubt can creep in, making you feel like you're just winging it where others seem to instinctively get it. But guess what? You're definitely not alone, and there are ways to combat it.
Recognize and Reflect
The first step to overcoming imposter syndrome is knowing when it's happening. Instead of brushing it under the rug, take a moment to acknowledge it. Reflect on your experiences and recognize that feeling like a fraud is surprisingly common. Many developers—even the ones you might look up to—have felt the same way.
Celebrate the Wins
It's easy to fixate on what's not working, but how about what's going right? Maybe you've cracked a tough algorithm or finally nailed down a tricky syntax. Set aside time to celebrate these wins, no matter how small they seem. Each victory adds to your toolkit, boosting your confidence in your coding prowess.
Find a Mentor or Tribe
Having a mentor or joining a coding group offers you a safety net. A mentor can offer advice, share their own experiences with overcoming challenges, and reassure you about your progress. Joining a community where you can share your hurdles and achievements can also provide a much-needed perspective. Knowing others have walked the same path makes a big difference.
Keep Learning and Practicing
Last but not least, keep sharpening your skills. Enroll in more coding classes, engage in coding challenges, or simply continue working on projects that interest you. Practicing over time not only hones your abilities but also fortifies your confidence, diminishing the grip of imposter syndrome over time.